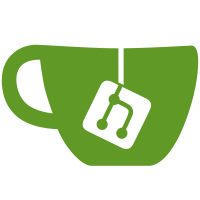
< Bubblegumdrop> SDLNet_UDP_Open seems to call SDLNet_Read16 (which is just SDL_SwapBE16) < Bubblegumdrop> But on the server side I don't use SDLNet_UDP_Open to host the port < whitt> probably the sender was flipping it but the receiver wasn't and so they were incompatible < Bubblegumdrop> removing the SDL_SwapBE16 on the client side fixed it
65 lines
1.3 KiB
C++
65 lines
1.3 KiB
C++
#include "debug.h"
|
|
#include "Client.h"
|
|
#include "UDP_Write.h"
|
|
|
|
#include <string>
|
|
|
|
int
|
|
main (int argc, char **argv)
|
|
{
|
|
int numsent;
|
|
const char *host = "localhost";
|
|
const Uint16 port = 6666;
|
|
const int channel = 1;
|
|
const size_t mtu = 1450;
|
|
Client c;
|
|
UDPsocket udpsock;
|
|
c = Client (host, port, channel);
|
|
if (!(udpsock = c.First ()))
|
|
{
|
|
SDL_Log ("Client error");
|
|
return 1;
|
|
}
|
|
|
|
std::string buf = "Hello, world!\n";
|
|
numsent = Buffer_Write_UDP (udpsock, channel, mtu, buf.c_str (), buf.length ());
|
|
|
|
SDL_Log ("%d", numsent);
|
|
c.UDP_CloseAll ();
|
|
|
|
return 0;
|
|
|
|
#if 0
|
|
IPaddress ipaddress;
|
|
UDPsocket udpsock;
|
|
UDPpacket *packet;
|
|
udpsock = SDLNet_UDP_Open (0);
|
|
if (SDLNet_ResolveHost (&ipaddress, host, port) < 0)
|
|
{
|
|
DEBUG_LOG ("SDLNet_ResolveHost: %s", SDLNet_GetError ());
|
|
return 0;
|
|
}
|
|
if (SDLNet_UDP_Bind (udpsock, channel, &ipaddress) < 0)
|
|
{
|
|
DEBUG_LOG ("SDLNet_UDP_Bind: %s", SDLNet_GetError ());
|
|
return 0;
|
|
}
|
|
|
|
packet = SDLNet_AllocPacket (1024);
|
|
if (packet)
|
|
{
|
|
packet->len = sprintf ((char*)packet->data, "Hello World!");
|
|
packet->address = ipaddress;
|
|
numsent = SDLNet_UDP_Send (udpsock, packet->channel, packet);
|
|
if (!numsent)
|
|
{
|
|
DEBUG_LOG ("SDLNet_UDP_Send: %s\n", SDLNet_GetError ());
|
|
}
|
|
}
|
|
|
|
SDLNet_UDP_Unbind (udpsock, channel);
|
|
SDLNet_UDP_Close (udpsock);
|
|
udpsock = NULL;
|
|
#endif
|
|
}
|