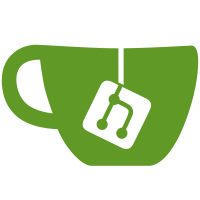
Another nuke! This time, trying to do a client <-> server thing. Also a bit of messing with Lua.
101 lines
1.4 KiB
C++
101 lines
1.4 KiB
C++
#include "FileIO.h"
|
|
|
|
#include <vector>
|
|
|
|
#include <errno.h> /* errno */
|
|
#include <stdio.h> /* FILE* */
|
|
#include <sys/stat.h> /* stat */
|
|
#include <sys/types.h> /* stat */
|
|
#include <unistd.h> /* stat */
|
|
|
|
FileIO::FileIO (const std::string& filename, const std::string& mode)
|
|
{
|
|
if (!(fp = Open (filename, mode)))
|
|
throw errno;
|
|
path = filename;
|
|
}
|
|
|
|
|
|
FILE*
|
|
FileIO::Open (const std::string& filename, const std::string& mode)
|
|
{
|
|
return fopen (filename.c_str (), mode.c_str ());
|
|
}
|
|
|
|
|
|
int
|
|
FileIO::Close (void)
|
|
{
|
|
return fclose (fp);
|
|
}
|
|
|
|
|
|
int
|
|
FileIO::Seek (const off_t offset, const int whence)
|
|
{
|
|
return fseeko (fp, offset, whence);
|
|
}
|
|
|
|
|
|
off_t
|
|
FileIO::Tell (void)
|
|
{
|
|
return ftello (fp);
|
|
}
|
|
|
|
|
|
size_t
|
|
FileIO::Read (void *dst, const size_t size)
|
|
{
|
|
return fread (dst, sizeof (char), size, fp);
|
|
}
|
|
|
|
|
|
size_t
|
|
FileIO::Write (const void *dst, const size_t size)
|
|
{
|
|
return fwrite (dst, sizeof (char), size, fp);
|
|
}
|
|
|
|
|
|
void
|
|
FileIO::Rewind (void)
|
|
{
|
|
rewind (fp);
|
|
}
|
|
|
|
|
|
time_t
|
|
FileIO::Mtime (void)
|
|
{
|
|
time_t rc;
|
|
struct stat sb;
|
|
rc = 0;
|
|
if (!stat (path.c_str (),&sb))
|
|
rc = sb.st_mtime;
|
|
else
|
|
throw errno;
|
|
return rc;
|
|
}
|
|
|
|
|
|
off_t
|
|
FileIO::Size (void)
|
|
{
|
|
off_t rc;
|
|
Seek (0, SEEK_END);
|
|
rc = Tell ();
|
|
Rewind ();
|
|
return rc;
|
|
}
|
|
|
|
|
|
std::string
|
|
FileIO::ReadToString (void)
|
|
{
|
|
off_t size = Size () + 1;
|
|
std::vector<char> b (size);
|
|
off_t nread = Read (b.data (), size);
|
|
return std::string ((const char *) b.data (), nread);
|
|
}
|