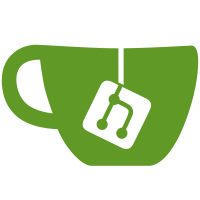
So when PACKET_SDL_EVENT was SDL_USEREVENT, things worked fine in the main loop it would see SDL_USEREVENT and send it off to my_SDL_UserEvent. But when I started adding more events, the SDL_UserEvent paradigm given in the SDL Wiki stopped working. The example here is broken: https://wiki.libsdl.org/SDL_AddTimer The example here is working and "correct": https://wiki.libsdl.org/SDL_UserEvent All SDL_RegisterEvents is increase a static counter. SDL doesn't actually use these events anyway. It's purely symbolic, as far as I can tell. So anyway. Now events work after a bunch of debugging. SHADER_PROGRAM_REFRESH is the second user event I've done. The ShaderProgram runs an SDL_TimerID that looks at mVertexPath, mGeometryPath if present, and mFragmentPath. mVertexPath and mFragmentPath are required by Compile3ShaderBuffers. In the future I'd like to change the way this class behaves.
74 lines
2.0 KiB
C++
74 lines
2.0 KiB
C++
#pragma once
|
|
|
|
#include <map>
|
|
#include <string>
|
|
|
|
#include <time.h>
|
|
#include <GL/glew.h>
|
|
|
|
#include <glm/glm.hpp>
|
|
#include <glm/gtc/type_ptr.hpp>
|
|
|
|
#include <SDL.h>
|
|
|
|
class ShaderProgram
|
|
{
|
|
/* TODO there's gotta be a better way of doing this! */
|
|
std::string mVertexPath, mGeometryPath, mFragmentPath;
|
|
SDL_TimerID mShaderWatchTimer;
|
|
/* most recent modification times for the three in order */
|
|
time_t mMtime[3];
|
|
|
|
bool mUniformLocationsNeedRefresh;
|
|
std::map<const std::string, GLint> UniformLocationCache;
|
|
|
|
static GLint my_checkCompileSuccess (const GLuint, const GLenum);
|
|
static Uint32 my_WatchShaderHelper (Uint32 interval, void* param);
|
|
|
|
GLint getCachedLoc (const std::string&);
|
|
|
|
public:
|
|
/*
|
|
* Set-able
|
|
*/
|
|
GLuint ID;
|
|
|
|
ShaderProgram (const std::string&, const std::string&, const std::string&);
|
|
|
|
~ShaderProgram (void);
|
|
|
|
/*
|
|
* gl* stuff. These modify the current `ID`.
|
|
*/
|
|
void RefreshUniformLocations (void);
|
|
void Use (void);
|
|
void Bool (const std::string&, const bool&);
|
|
void Float (const std::string&, const float&);
|
|
void Int (const std::string&, const int&);
|
|
void Vec2 (const std::string&, const glm::vec2&);
|
|
void Vec3 (const std::string&, const glm::vec3&);
|
|
void Vec4 (const std::string&, const glm::vec4&);
|
|
void Mat2 (const std::string&, const glm::mat2&);
|
|
void Mat3 (const std::string&, const glm::mat3&);
|
|
void Mat4 (const std::string&, const glm::mat4&);
|
|
|
|
/*
|
|
* Program/util stuff
|
|
*/
|
|
GLuint CompileShaderBuffer (const std::string&, const GLenum);
|
|
GLuint Compile3ShaderBuffers (const std::string&, const std::string&, const std::string&);
|
|
GLuint CompileShaderPath (const std::string&, const GLenum);
|
|
GLint LinkProgram (void);
|
|
|
|
/*
|
|
* Monitor the modification time of the loaded shaders
|
|
*/
|
|
void StartWatchingShader (void);
|
|
void StopWatchingShader (void);
|
|
bool NeedsReloading (bool);
|
|
|
|
const std::string& getVertexPath (void) const { return mVertexPath; }
|
|
const std::string& getGeometryPath (void) const { return mGeometryPath; }
|
|
const std::string& getFragmentPath (void) const { return mFragmentPath; }
|
|
};
|