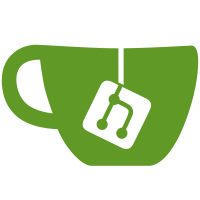
Another nuke! This time, trying to do a client <-> server thing. Also a bit of messing with Lua.
90 lines
1.5 KiB
C++
90 lines
1.5 KiB
C++
#include "FileIO.h"
|
|
#include "UDP_Write.h"
|
|
|
|
#include <vector>
|
|
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <stdlib.h>
|
|
#include <getopt.h>
|
|
#include <unistd.h>
|
|
|
|
#include <SDL_net.h>
|
|
|
|
static void usage (const char* argv0)
|
|
{
|
|
fprintf (stderr, "Usage: %s [-f path] [-h] [-p port] [-s server]\n", argv0);
|
|
}
|
|
|
|
int
|
|
main (int argc, char** argv)
|
|
{
|
|
int opt;
|
|
int numsent;
|
|
int mtu;
|
|
int channel;
|
|
const char* path;
|
|
const char* server;
|
|
Uint16 port;
|
|
|
|
path = NULL;
|
|
server = "localhost";
|
|
port = 6666;
|
|
channel = 1;
|
|
mtu = 1450;
|
|
numsent = 0;
|
|
|
|
while ((opt = getopt (argc, argv, "hs:p:f:")) != -1)
|
|
{
|
|
switch (opt)
|
|
{
|
|
case 'h':
|
|
usage (argv[0]);
|
|
exit (EXIT_FAILURE);
|
|
break;
|
|
case 's':
|
|
server = optarg;
|
|
if (!strcmp (server, "NULL"))
|
|
server = NULL;
|
|
break;
|
|
case 'p':
|
|
port = strtol (optarg, NULL, 0);
|
|
break;
|
|
case 'f':
|
|
path = optarg;
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
}
|
|
|
|
#if 0
|
|
if (optind < argc) {
|
|
printf("non-option ARGV-elements: ");
|
|
while (optind < argc)
|
|
printf("%s ", argv[optind++]);
|
|
printf("\n");
|
|
}
|
|
#endif
|
|
|
|
if ((optind < argc) && (argv[optind][0] == '-'))
|
|
{
|
|
const size_t size = 8192;
|
|
std::vector<char> buf (size);
|
|
std::fill (buf.begin(), buf.end(), 0);
|
|
size_t nread = fread (buf.data (), sizeof (char), size - 1, stdin);
|
|
numsent = SendBuffer_UDP (server, port, channel, mtu, buf.data (), nread);
|
|
}
|
|
else
|
|
{
|
|
if (path)
|
|
{
|
|
numsent = SendFile_UDP (server, port, channel, mtu, path);
|
|
}
|
|
}
|
|
|
|
SDL_Log ("%d", numsent);
|
|
|
|
return 0;
|
|
}
|