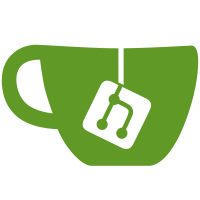
Server listens correctly with threads and stdin reader thread. Client does nothing currently gutting UDP_Write for a UDPbase class introduced in this commit.
44 lines
1.2 KiB
C++
44 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "lib_lua_common.h"
|
|
#include "UDPbase.h"
|
|
|
|
class Server : public UDPbase
|
|
{
|
|
SDL_mutex* mProcessPacketMutex;
|
|
SDL_TimerID mFetchPacketsCallbackTimerID;
|
|
|
|
std::vector<TCPsocket> TCPsocks;
|
|
std::vector<UDPsocket> UDPsocks;
|
|
UDPpacket** UDPPacketV;
|
|
SDLNet_SocketSet SocketSet;
|
|
lua_State *mLuaState;
|
|
|
|
public:
|
|
Server (const char* host, const Uint16 port);
|
|
~Server (void);
|
|
|
|
static Uint32 FetchPacketsCallback (Uint32 interval, void* param);
|
|
void ProcessPacketHelper (UDPpacket* packet);
|
|
void RunLua (const std::string& buf);
|
|
int AllocateApproximateBufferSpace (const int nclients);
|
|
int Start (void);
|
|
int Stop (void);
|
|
int Lock (void)
|
|
{
|
|
return SDL_LockMutex (mProcessPacketMutex);
|
|
}
|
|
int Unlock (void)
|
|
{
|
|
return SDL_UnlockMutex (mProcessPacketMutex);
|
|
}
|
|
lua_State* getLuaState(void) const { return mLuaState; }
|
|
const TCPsocket* getTCPsock (size_t idx) const { return &TCPsocks.at (idx); }
|
|
const UDPsocket* getUDPsock (size_t idx) const { return &UDPsocks.at (idx); }
|
|
UDPpacket* getUDPPacketV (size_t idx) const { return UDPPacketV[idx]; }
|
|
SDLNet_SocketSet getSocketSet (void) const { return SocketSet; }
|
|
};
|