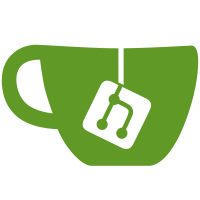
Quite a bit going on in here. - Messing with shaders - Move ShaderProgram to std::unique_ptr These are part of efforts to clean up the ShaderProgram class to have a better interface. - Working on shell-ing out commands. This is pointless but getting me in the state of thinking about the system as a whole - e.g. "stop" at the "prompt" should "stop the server," so the prompt thread will need to communicate with the main thread. We already have event signalling in place for this. - Triangle strip cube Performance testing reasons. Not being used for now. - Remove TCP stuff from UDPbase. It only does UDP now.
53 lines
1.2 KiB
C++
53 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "lib_lua_common.h"
|
|
#include "UDPbase.h"
|
|
|
|
#define DEFAULT_SOCKET_TIMEOUT 10
|
|
|
|
class Server : public UDPbase
|
|
{
|
|
SDL_mutex* mProcessPacketMutex;
|
|
SDL_TimerID mFetchPacketsCallbackTimerID;
|
|
|
|
SDLNet_SocketSet SocketSet;
|
|
UDPpacket** packetV;
|
|
lua_State *mLuaState;
|
|
|
|
public:
|
|
Server (const char* host, const Uint16 port);
|
|
~Server (void);
|
|
|
|
/*
|
|
* error: invalid use of non-static member function Uint32 Server::FetchPacketsCallback(Uint32, void*)
|
|
* Don't understand this.
|
|
* static keyword fixes it.
|
|
*/
|
|
static Uint32 FetchPacketsCallback (Uint32 interval, void* param);
|
|
|
|
void ProcessPacketHelper (UDPpacket* packet);
|
|
|
|
void RunLua (const std::string& buf);
|
|
|
|
int AllocateApproximateResources (const int nclients);
|
|
|
|
int Start (void);
|
|
int Stop (void);
|
|
int Lock (void)
|
|
{
|
|
return SDL_LockMutex (mProcessPacketMutex);
|
|
}
|
|
int Unlock (void)
|
|
{
|
|
return SDL_UnlockMutex (mProcessPacketMutex);
|
|
}
|
|
|
|
lua_State* getLuaState(void) { return mLuaState; }
|
|
UDPsocket getUDPsock (size_t idx) { return UDPsocks.at (idx); }
|
|
UDPpacket** getPacketV (void) { return packetV; }
|
|
SDLNet_SocketSet getSocketSet (void) { return SocketSet; }
|
|
};
|