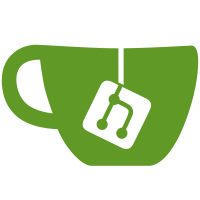
Quite a bit going on in here. - Messing with shaders - Move ShaderProgram to std::unique_ptr These are part of efforts to clean up the ShaderProgram class to have a better interface. - Working on shell-ing out commands. This is pointless but getting me in the state of thinking about the system as a whole - e.g. "stop" at the "prompt" should "stop the server," so the prompt thread will need to communicate with the main thread. We already have event signalling in place for this. - Triangle strip cube Performance testing reasons. Not being used for now. - Remove TCP stuff from UDPbase. It only does UDP now.
37 lines
895 B
C++
37 lines
895 B
C++
#pragma once
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include <SDL_net.h>
|
|
|
|
#define DEFAULT_MAX_PACKET_SIZE 250
|
|
|
|
class UDPbase
|
|
{
|
|
int mChannel;
|
|
IPaddress mIPAddress;
|
|
protected:
|
|
std::vector<UDPsocket> UDPsocks;
|
|
public:
|
|
UDPbase (const char* host, const Uint16 port);
|
|
UDPbase (const char* host, const Uint16 port, const int channel);
|
|
~UDPbase (void);
|
|
|
|
int ResolveHost (IPaddress*, const char*, const Uint16);
|
|
|
|
UDPsocket UDP_Open (void);
|
|
UDPsocket UDP_Open (const Uint16 port);
|
|
void UDP_Close (UDPsocket sock);
|
|
int UDP_Bind (UDPsocket sock, const int channel);
|
|
void UDP_Unbind (UDPsocket sock, const int channel);
|
|
IPaddress* UDP_GetPeerAddress (UDPsocket sock, const int channel);
|
|
|
|
void CloseAllSockets (void);
|
|
|
|
int getChannel (void) const { return mChannel; }
|
|
UDPsocket getSocket (const size_t idx) const { return UDPsocks.at (idx); }
|
|
|
|
std::string toString (void);
|
|
};
|