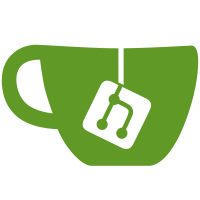
Quite a bit going on in here. - Messing with shaders - Move ShaderProgram to std::unique_ptr These are part of efforts to clean up the ShaderProgram class to have a better interface. - Working on shell-ing out commands. This is pointless but getting me in the state of thinking about the system as a whole - e.g. "stop" at the "prompt" should "stop the server," so the prompt thread will need to communicate with the main thread. We already have event signalling in place for this. - Triangle strip cube Performance testing reasons. Not being used for now. - Remove TCP stuff from UDPbase. It only does UDP now.
61 lines
1.2 KiB
C++
61 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include <SDL_net.h>
|
|
|
|
/**
|
|
|
|
# UDPpacketVBuffer
|
|
|
|
Create your `UDPsocket` somewhere:
|
|
|
|
UDPsocket sock;
|
|
...
|
|
|
|
You have a buffer:
|
|
|
|
FileIO f (path, "r");
|
|
std::string buf = f.ReadToString ();
|
|
|
|
Allocate a setup packet and set `len` to your MTU, set your destination `address`, and the destination `channel`.
|
|
I'm not sure -1 will work as a channel, be sure to set it to your active channel.
|
|
|
|
UDPpacket* packet = SDL_AllocPacket (buf.length ());
|
|
packet->len = DEFAULT_MAX_PACKET_SIZE;
|
|
packet->channel = -1;
|
|
packet->address = GetPeerAddress (sock, packet->channel);
|
|
|
|
Use `UDPpacketVBuffer` wrapper to send the data, the destructor will automatically free the `UDPpacket**`:
|
|
|
|
UDPpacketVBuffer packetV (&buf[0], buf.length ());
|
|
packetV.Send (sock);
|
|
|
|
Then free the leftover setup packet:
|
|
|
|
SDLNet_FreePacket (packet);
|
|
|
|
*/
|
|
class UDPpacketVBuffer
|
|
{
|
|
int npackets;
|
|
UDPpacket** packetV;
|
|
public:
|
|
UDPpacketVBuffer (UDPpacket* src, const void* buf, const size_t size);
|
|
~UDPpacketVBuffer (void)
|
|
{
|
|
if (packetV)
|
|
{
|
|
SDLNet_FreePacketV (packetV);
|
|
packetV = NULL;
|
|
}
|
|
}
|
|
int Send (UDPsocket sock)
|
|
{
|
|
int numsent = 0;
|
|
if (packetV)
|
|
{
|
|
numsent = SDLNet_UDP_SendV (sock, packetV, npackets);
|
|
}
|
|
return numsent;
|
|
}
|
|
};
|